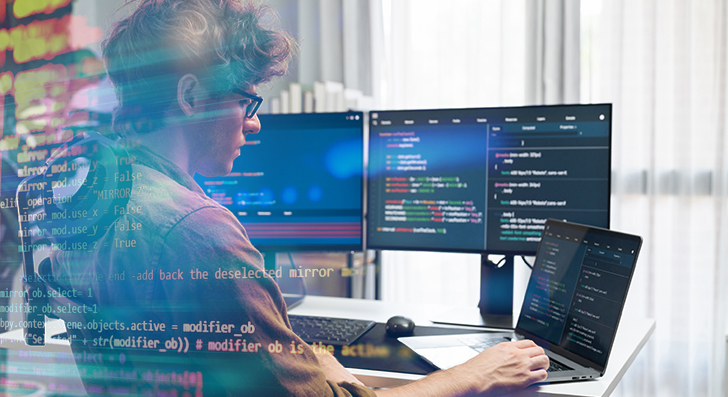
Scalability usually means your application can handle advancement—additional consumers, much more details, plus more website traffic—with no breaking. To be a developer, constructing with scalability in mind will save time and anxiety afterwards. Below’s a clear and simple guidebook that may help you commence by Gustavo Woltmann.
Design for Scalability from the beginning
Scalability isn't a thing you bolt on later—it should be aspect of the prepare from the beginning. A lot of applications fall short when they increase fast mainly because the original layout can’t take care of the additional load. Like a developer, you might want to Feel early regarding how your system will behave under pressure.
Get started by creating your architecture to be versatile. Stay clear of monolithic codebases exactly where all the things is tightly connected. Alternatively, use modular structure or microservices. These patterns split your application into smaller sized, impartial pieces. Every module or provider can scale By itself without affecting The entire process.
Also, consider your databases from working day one. Will it require to manage 1,000,000 buyers or just a hundred? Choose the proper type—relational or NoSQL—according to how your info will increase. System for sharding, indexing, and backups early, Even when you don’t need them however.
Yet another critical issue is to avoid hardcoding assumptions. Don’t create code that only functions below existing problems. Think of what would transpire If the person foundation doubled tomorrow. Would your application crash? Would the databases decelerate?
Use layout designs that help scaling, like concept queues or occasion-driven systems. These aid your application cope with additional requests devoid of receiving overloaded.
If you Create with scalability in mind, you're not just preparing for success—you are lowering potential headaches. A well-planned system is less complicated to take care of, adapt, and increase. It’s greater to get ready early than to rebuild later on.
Use the correct Database
Choosing the right databases can be a crucial Portion of developing scalable applications. Not all databases are built the same, and utilizing the Improper you can gradual you down or maybe lead to failures as your app grows.
Get started by knowledge your details. Could it be very structured, like rows inside of a desk? If Sure, a relational databases like PostgreSQL or MySQL is an efficient in good shape. They are powerful with interactions, transactions, and regularity. In addition they aid scaling procedures like go through replicas, indexing, and partitioning to handle far more visitors and info.
In case your information is more flexible—like person exercise logs, solution catalogs, or files—take into account a NoSQL option like MongoDB, Cassandra, or DynamoDB. NoSQL databases are greater at handling massive volumes of unstructured or semi-structured facts and can scale horizontally additional effortlessly.
Also, look at your read and publish styles. Have you been accomplishing plenty of reads with much less writes? Use caching and browse replicas. Are you dealing with a major publish load? Explore databases that can take care of significant produce throughput, or perhaps function-primarily based knowledge storage units like Apache Kafka (for short-term knowledge streams).
It’s also clever to think ahead. You may not want State-of-the-art scaling options now, but choosing a databases that supports them suggests you received’t require to change later on.
Use indexing to hurry up queries. Stay clear of unnecessary joins. Normalize or denormalize your info dependant upon your obtain styles. And generally check databases general performance when you grow.
In a nutshell, the right database is determined by your application’s structure, pace desires, and how you assume it to mature. Consider time to choose properly—it’ll help you save lots of problems later on.
Improve Code and Queries
Quickly code is vital to scalability. As your app grows, every single modest delay adds up. Poorly penned code or unoptimized queries can decelerate functionality and overload your technique. That’s why it’s vital that you Make productive logic from the start.
Commence by creating clean, very simple code. Prevent repeating logic and remove something avoidable. Don’t select the most sophisticated Resolution if a simple a person will work. Maintain your capabilities small, targeted, and easy to check. Use profiling resources to uncover bottlenecks—sites wherever your code can take also long to operate or utilizes far too much memory.
Up coming, look at your databases queries. These often sluggish things down in excess of the code itself. Make sure Every single question only asks for the information you truly require. Prevent Choose *, which fetches all the things, and as an alternative pick out particular fields. Use indexes to hurry up lookups. And avoid undertaking too many joins, Specially throughout big tables.
When you notice precisely the same details becoming requested time and again, use caching. Store the outcome quickly making use of instruments like Redis or Memcached so you don’t must repeat high priced functions.
Also, batch your databases operations whenever you can. In place of updating a row one after the other, update them in teams. This cuts down on overhead and tends to make your app far more efficient.
Remember to examination with massive datasets. Code and queries that do the job fine with 100 information may well crash whenever they have to manage one million.
To put it briefly, scalable applications are speedy applications. Keep your code tight, your queries lean, and use caching when necessary. These methods support your software keep clean and responsive, whilst the load boosts.
Leverage Load Balancing and Caching
As your application grows, it's to deal with far more end users plus more traffic. If everything goes through one server, it will quickly turn into a bottleneck. That’s where by load balancing and caching are available. Both of these equipment aid keep your app speedy, stable, and scalable.
Load balancing spreads incoming traffic throughout many servers. As an alternative to one particular server carrying out all of the function, the load balancer routes users to distinctive servers dependant on availability. This suggests no one server will get overloaded. If 1 server goes down, the load balancer can ship traffic to the Many others. Instruments like Nginx, HAProxy, or cloud-based mostly options from AWS and Google Cloud make this straightforward to put in place.
Caching is about storing info quickly so it could be reused swiftly. When users ask for the identical information yet again—like an item webpage or a profile—you don’t should fetch it in the databases each and every time. You can provide it in the cache.
There's two typical different types of caching:
1. Server-facet caching (like Redis or Memcached) retailers details in memory for quick obtain.
2. Customer-side caching (like browser caching or CDN caching) merchants static files near to the person.
Caching decreases databases load, improves pace, and makes your app extra productive.
Use caching for things which don’t adjust often. And constantly be certain your cache is up to date when facts does alter.
In a nutshell, load balancing and caching are simple but effective applications. With each other, they help your application deal with far more buyers, keep speedy, and recover from troubles. If you propose to grow, you will need both equally.
Use Cloud and Container Tools
To construct scalable apps, you require tools that let your app expand quickly. That’s where by cloud platforms and containers come in. They provide you overall flexibility, cut down set up time, and make scaling much smoother.
Cloud platforms like Amazon Net Companies (AWS), Google Cloud System (GCP), and Microsoft Azure Enable you to lease servers and expert services as you would like them. You don’t get more info have to purchase hardware or guess long term capability. When site visitors will increase, you could increase more resources with just some clicks or automatically using vehicle-scaling. When targeted visitors drops, you can scale down to economize.
These platforms also give products and services like managed databases, storage, load balancing, and stability instruments. You may center on constructing your app rather than controlling infrastructure.
Containers are One more crucial Instrument. A container packages your application and almost everything it has to run—code, libraries, configurations—into just one unit. This makes it simple to maneuver your application among environments, from your notebook to your cloud, without the need of surprises. Docker is the most well-liked Instrument for this.
When your application makes use of multiple containers, applications like Kubernetes allow you to control them. Kubernetes handles deployment, scaling, and Restoration. If one particular component within your application crashes, it restarts it immediately.
Containers also enable it to be simple to separate portions of your app into products and services. You could update or scale areas independently, that is perfect for overall performance and trustworthiness.
In brief, applying cloud and container equipment means it is possible to scale fast, deploy simply, and recover speedily when problems come about. If you want your app to mature without having restrictions, start off using these equipment early. They help you save time, decrease possibility, and help you remain centered on building, not repairing.
Watch Everything
Should you don’t watch your software, you won’t know when items go Erroneous. Checking assists you see how your application is accomplishing, place troubles early, and make improved decisions as your app grows. It’s a crucial Component of setting up scalable methods.
Commence by tracking primary metrics like CPU use, memory, disk House, and reaction time. These let you know how your servers and providers are undertaking. Instruments like Prometheus, Grafana, Datadog, or New Relic will let you collect and visualize this information.
Don’t just keep track of your servers—check your app much too. Regulate how much time it's going to take for users to load pages, how often errors occur, and exactly where they happen. Logging resources like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will help you see what’s taking place inside your code.
Setup alerts for important problems. For instance, In case your response time goes higher than a Restrict or maybe a assistance goes down, you must get notified straight away. This allows you deal with difficulties rapidly, typically just before customers even notice.
Checking is likewise valuable once you make modifications. If you deploy a completely new element and see a spike in errors or slowdowns, you could roll it back again prior to it causes authentic hurt.
As your app grows, targeted visitors and knowledge enhance. With out checking, you’ll overlook signs of issues until finally it’s too late. But with the appropriate resources set up, you remain on top of things.
In a nutshell, checking will help you keep your app reliable and scalable. It’s not almost spotting failures—it’s about comprehension your method and making certain it works properly, even under pressure.
Ultimate Views
Scalability isn’t just for big corporations. Even little applications need a robust Basis. By developing diligently, optimizing properly, and utilizing the ideal equipment, you could Construct applications that grow easily without the need of breaking under pressure. Start off small, Feel major, and Develop sensible.