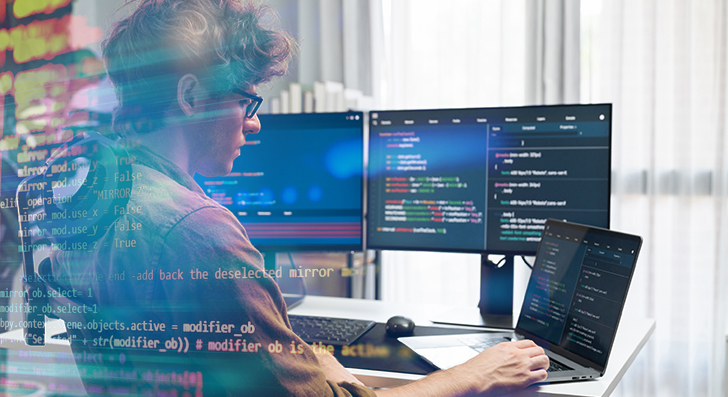
Scalability means your application can manage development—more people, far more info, plus more targeted visitors—devoid of breaking. Like a developer, building with scalability in your mind saves time and worry later on. Here’s a clear and simple guidebook that will help you begin by Gustavo Woltmann.
Structure for Scalability from the Start
Scalability is just not anything you bolt on afterwards—it ought to be portion of your prepare from the beginning. A lot of applications fall short when they increase fast because the initial structure can’t manage the additional load. As being a developer, you'll want to Believe early regarding how your system will behave stressed.
Begin by planning your architecture to be versatile. Prevent monolithic codebases exactly where almost everything is tightly related. Rather, use modular style and design or microservices. These patterns split your application into smaller, impartial components. Just about every module or service can scale on its own with no affecting The entire process.
Also, think about your database from day one particular. Will it have to have to handle a million consumers or just a hundred? Choose the proper variety—relational or NoSQL—dependant on how your data will develop. Program for sharding, indexing, and backups early, Even though you don’t need to have them however.
One more significant place is to stay away from hardcoding assumptions. Don’t write code that only works below present situations. Think of what would happen if your user foundation doubled tomorrow. Would your app crash? Would the databases decelerate?
Use structure styles that guidance scaling, like information queues or event-pushed units. These assistance your application take care of far more requests with no receiving overloaded.
If you Construct with scalability in mind, you're not just preparing for success—you might be cutting down foreseeable future head aches. A effectively-planned procedure is less complicated to take care of, adapt, and improve. It’s greater to organize early than to rebuild later.
Use the ideal Databases
Selecting the right databases can be a crucial Section of developing scalable applications. Not all databases are crafted the exact same, and using the wrong you can slow you down or simply bring about failures as your application grows.
Commence by comprehending your details. Could it be highly structured, like rows in a very table? If Certainly, a relational database like PostgreSQL or MySQL is a good in shape. These are typically sturdy with relationships, transactions, and consistency. In addition they assist scaling methods like examine replicas, indexing, and partitioning to handle additional site visitors and details.
If the info is a lot more flexible—like consumer exercise logs, solution catalogs, or files—contemplate a NoSQL possibility like MongoDB, Cassandra, or DynamoDB. NoSQL databases are far better at managing massive volumes of unstructured or semi-structured details and may scale horizontally additional very easily.
Also, take into consideration your study and produce styles. Have you been accomplishing plenty of reads with much less writes? Use caching and read replicas. Have you been managing a heavy compose load? Check into databases that can manage substantial generate throughput, or perhaps function-based information storage techniques like Apache Kafka (for momentary details streams).
It’s also smart to Feel forward. You may not will need Highly developed scaling features now, but choosing a databases that supports them suggests you received’t have to have to modify later.
Use indexing to hurry up queries. Avoid pointless joins. Normalize or denormalize your info according to your entry styles. And always monitor database overall performance as you develop.
In brief, the correct database depends upon your app’s structure, velocity wants, And the way you count on it to develop. Consider time to pick wisely—it’ll help you save loads of issues later on.
Enhance Code and Queries
Quick code is vital to scalability. As your app grows, each small hold off provides up. Badly created code or unoptimized queries can decelerate general performance and overload your process. That’s why it’s crucial that you Construct successful logic from the start.
Begin by crafting cleanse, basic code. Stay away from repeating logic and remove nearly anything unneeded. Don’t choose the most elaborate Option if an easy a single functions. Keep the features brief, concentrated, and simple to check. Use profiling instruments to locate bottlenecks—sites the place your code requires much too extensive to run or uses an excessive amount memory.
Up coming, evaluate your database queries. These normally sluggish matters down a lot more than the code alone. Be sure each question only asks for the information you truly require. Prevent Choose *, which fetches all the things, and rather pick out particular fields. Use indexes to hurry up lookups. And avoid carrying out a lot of joins, Specially throughout big tables.
When you notice precisely the same details becoming asked for many times, use caching. Shop the outcome quickly using resources like Redis or Memcached so that you don’t really need to repeat high priced operations.
Also, batch your database operations any time you can. In lieu of updating a row one by one, update them in groups. This cuts down on overhead and helps make your application additional economical.
Remember to examination with substantial datasets. Code and queries that work good with 100 information may possibly crash if they have to take care of one million.
To put it briefly, scalable applications are fast apps. Keep your code restricted, your queries lean, and use caching when essential. These techniques assistance your software continue to be sleek and responsive, at the same time as the load improves.
Leverage Load Balancing and Caching
As your application grows, it's got to take care of extra buyers and more traffic. If almost everything goes by way of one particular server, it is going to speedily become a bottleneck. That’s in which load balancing and caching are available in. These two tools assistance keep the application rapidly, steady, and scalable.
Load balancing spreads incoming visitors across numerous servers. Rather than 1 server performing all the do the job, the load balancer routes people to unique servers determined by availability. This implies no single server receives overloaded. If one particular server goes down, the load balancer can deliver visitors to the Other people. Tools like Nginx, HAProxy, or cloud-centered remedies from AWS and Google Cloud make this simple to set up.
Caching is about storing details briefly so it can be reused immediately. When end users request a similar data once more—like an item website page or possibly a profile—you don’t must fetch it from the databases each time. You could serve it within the cache.
There are 2 common sorts of caching:
1. Server-aspect caching (like Redis or Memcached) shops facts in memory for quickly obtain.
two. Client-aspect caching (like browser caching or CDN caching) stores static documents close to the consumer.
Caching cuts down database load, increases speed, and can make your app far more economical.
Use caching for things that don’t transform typically. And always be sure your cache is updated when knowledge does improve.
In brief, load balancing and caching are very simple but potent instruments. Together, they help your application tackle much more end users, continue to be quick, and Get better from issues. If you intend to improve, you need the two.
Use Cloud and Container Instruments
To build scalable applications, you may need instruments that permit your application develop very easily. That’s wherever cloud platforms and containers are available. They offer you flexibility, lessen set up time, and make scaling A great deal smoother.
Cloud platforms like Amazon Web Providers (AWS), Google Cloud Platform (GCP), and Microsoft Azure Allow you to lease servers and companies as you would like them. You don’t really have to buy hardware or guess future capacity. When visitors raises, you'll be able to incorporate more resources with just a few clicks or automatically using auto-scaling. When traffic drops, you can scale down to save money.
These platforms also offer services like managed databases, storage, load balancing, and stability applications. You could center on making your application as opposed to handling infrastructure.
Containers are An additional essential Device. A container deals your app and everything it really should operate—code, libraries, options—into 1 device. This causes it to be straightforward to move your application amongst environments, out of your laptop to your cloud, with no surprises. Docker is the most well-liked tool for this.
When your application employs numerous containers, applications like Kubernetes make it easier to deal with them. Kubernetes handles deployment, scaling, and recovery. If a person portion of one's application crashes, it restarts it routinely.
Containers also make it straightforward to independent parts of your application into solutions. You could update or scale areas independently, which can be great for functionality and reliability.
Briefly, utilizing cloud and container applications implies you could scale quickly, deploy easily, and Recuperate immediately when difficulties materialize. If you need your application to develop devoid of limits, start off using these applications early. They conserve time, lower risk, and allow you to continue to be focused on creating, not correcting.
Monitor Almost everything
For those who don’t keep track of your application, you gained’t know when matters go Incorrect. Monitoring can help the thing is how your app is executing, place challenges early, and make far better selections as your application grows. It’s a important Portion of making scalable units.
Begin by tracking simple metrics like CPU utilization, memory, disk Place, and reaction time. These show you how your servers and services are performing. Equipment like Prometheus, Grafana, Datadog, or New Relic may help you accumulate and visualize this details.
Don’t just observe your servers—monitor your app as well. Keep an eye on how much time it will require for buyers to load internet pages, how frequently faults materialize, and where by they occur. Logging tools like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can help you see what’s happening within your code.
Arrange alerts for vital complications. Such as, In the event your reaction time goes earlier mentioned a Restrict or possibly a support goes down, you ought to get notified right away. This assists you correct concerns quickly, frequently before users even see.
Checking is additionally helpful when you make variations. When you deploy a whole new characteristic and see a spike in faults or slowdowns, it is possible to roll it back before it will cause true harm.
As your application grows, targeted traffic and info increase. Devoid of checking, you’ll skip indications of difficulties till it’s much too late. But with the best tools set up, you stay on top of things.
In brief, checking aids you keep the app responsible and scalable. It’s not nearly recognizing failures—it’s about comprehension your system and making certain it really works properly, even stressed.
Ultimate Views
Scalability isn’t just for major businesses. Even smaller applications need a robust Basis. By developing diligently, optimizing properly, and utilizing the right equipment, you can Construct applications that more info grow efficiently without the need of breaking under pressure. Start off compact, Believe major, and build wise.